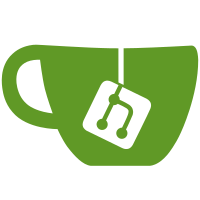
In case there are any future libraries written for Roblox in C#, I want to avoid running into any namespace collisions. Its best to keep everything pertaining to this project nested in its own unique namespace.
48 lines
1.1 KiB
C#
48 lines
1.1 KiB
C#
namespace RobloxFiles.DataTypes
|
|
{
|
|
public struct Ray
|
|
{
|
|
public readonly Vector3 Origin;
|
|
public readonly Vector3 Direction;
|
|
|
|
public Ray Unit
|
|
{
|
|
get
|
|
{
|
|
Ray unit;
|
|
|
|
if (Direction.Magnitude == 1.0f)
|
|
unit = this;
|
|
else
|
|
unit = new Ray(Origin, Direction.Unit);
|
|
|
|
return unit;
|
|
}
|
|
}
|
|
|
|
public Ray(Vector3 origin, Vector3 direction)
|
|
{
|
|
Origin = origin;
|
|
Direction = direction;
|
|
}
|
|
|
|
public override string ToString()
|
|
{
|
|
return '{' + Origin.ToString() + "}, {" + Direction.ToString() + '}';
|
|
}
|
|
|
|
public Vector3 ClosestPoint(Vector3 point)
|
|
{
|
|
Vector3 offset = point - Origin;
|
|
float diff = offset.Dot(Direction) / Direction.Dot(Direction);
|
|
return Origin + (diff * Direction);
|
|
}
|
|
|
|
public float Distance(Vector3 point)
|
|
{
|
|
Vector3 projected = ClosestPoint(point);
|
|
return (point - projected).Magnitude;
|
|
}
|
|
}
|
|
}
|