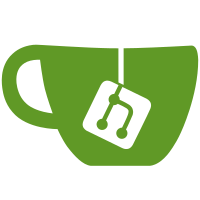
There's a lot of code at play here, so I haven't tested it yet. A good chunk of the components are available though.
30 lines
764 B
C#
30 lines
764 B
C#
using System;
|
|
using Roblox.Enums;
|
|
|
|
namespace Roblox.DataTypes
|
|
{
|
|
public struct PathWaypoint
|
|
{
|
|
public readonly Vector3 Position;
|
|
public readonly PathWaypointAction Action;
|
|
|
|
public PathWaypoint(Vector3? position)
|
|
{
|
|
Position = position ?? Vector3.Zero;
|
|
Action = PathWaypointAction.Walk;
|
|
}
|
|
|
|
public PathWaypoint(Vector3 position, PathWaypointAction action = PathWaypointAction.Walk)
|
|
{
|
|
Position = position;
|
|
Action = action;
|
|
}
|
|
|
|
public override string ToString()
|
|
{
|
|
Type PathWaypointAction = typeof(PathWaypointAction);
|
|
return '{' + Position + "} " + Enum.GetName(PathWaypointAction, Action);
|
|
}
|
|
}
|
|
}
|